공부 정리 블로그
변수 ~ 함수 본문
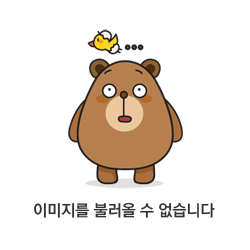
물리적으로 공간을 차지하는 것 - 실존함
컴퓨터에서 존재한다 / 존재하지 않는다를 어떻게 구별?
존재한다 -> 메모리에 저장 돼 있는 정보 -> 객체
정보 / 값 / 숫자 등 의미 있는 것들을 메모리에 저장 -> 객체
변수란?
객체는 메모리에 담겨 있음, 객체를 메모리에 담고 싶은데
담겨 있는 것을 사용하기 위해서는 이름이 있어야 한다.
숫자 주소 대신 문자로 된 이름을 붙여줌 -> 프로그래머는 숫자로 된 주소를 다르기 어렵기 때문에 사람이 인식 할 수 있도록 이름을 달아 줌
#include <iostream>
int main()
{
// x라는 정수형 변수를 선언했다.
// x라는 메모리 공간을 선언
// 크기는 123을 담을 수 있을 만큼있음
// initialization (cf. assignment)
int x = 123;
int y;
// assignment (임무를 할당한다.)
// '='는 같다라는 의미가 아님
// y라는 변수 이름이 가리키고 있는 메모리 공간에 '123'이라는 정수를 저장한다
y = 123;
std::cout << x << std::endl;
std::cout << &x << std::endl;
return 0;
}
질문 할 것
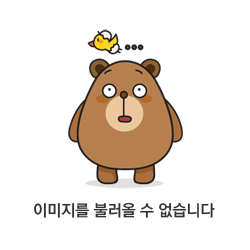
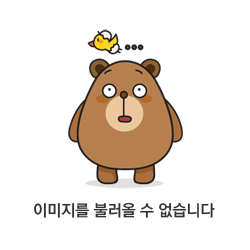
강의에서는 주소가 나오는데, 나는 안 나옴 ㅠ
아 디버깅 모드라서 그랬음
release mode 어케 키나요 ? 혹시 디버깅 없이 시작?
#include <iostream>
int main()
{
int x = 1;
x = x + 2;
std::cout << x << std::endl; // #1
int y = x;
std::cout << y << std::endl; //#2
// is (x + y) 1-value or r-value?
std::cout << x + y << std::endl;//#3
std::cout << x << std::endl; //#4
int z;
std::cout << z << std::endl; //#5
return 0;
}
2
6
3
1
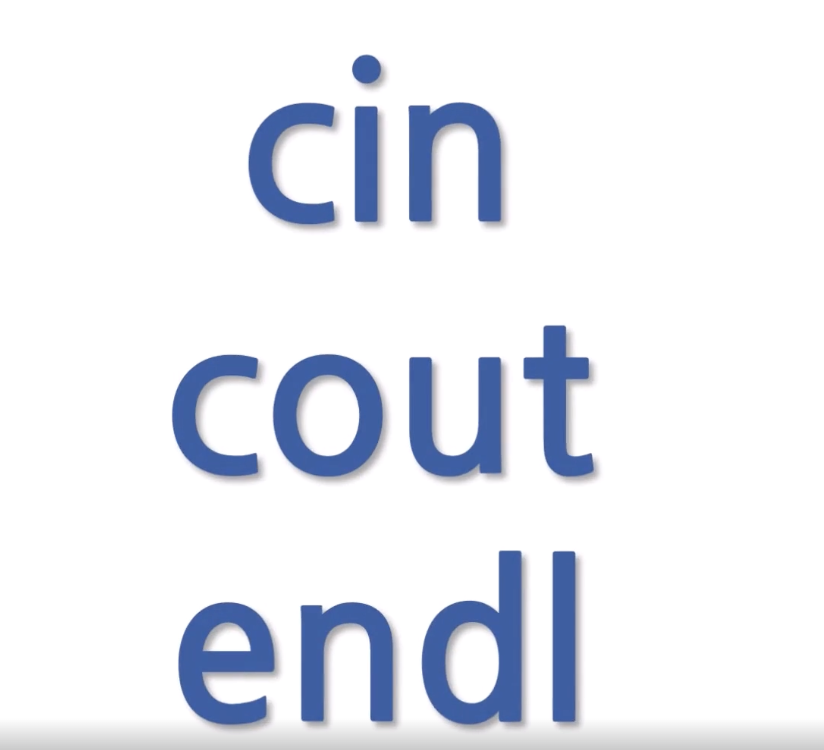
#include <iostream> //cout, cin, endl, ...
#include <cstdio> // printf
int main()
{
//이 줄을 쓰면 아래와 같이 사용 가능
// using namespace std;
//cout << "x is" << x << "pi is " << pi << endl;
int x = 1024;
double pi = 3.141592;
std::cout << "I love this lecture!\n"; // << std::endl;
std::cout << "x is" << x << "pi is " << pi << std::endl;
std::cout<< "abc" << "\t" <<"def" << std::endl;
std::cout<< "ab" << "\t" <<"cdef" << std::endl;
return 0;
}
I love this lecture!
x is1024pi is 3.14159
abc def
ab cdef
#include <iostream> //cout, cin, endl, ...
#include <cstdio> // printf
int main()
{
using namespace std;
int x = 1024;
double pi = 3.141592;
cout << "I love this lecture!\n"; // << std::endl;
cout << "x is" << x << "pi is " << pi << endl;
cout<< "abc" << "\t" <<"def" << endl;
cout<< "ab" << "\t" <<"cdef" << endl;
//mac에서는 오디오 출력 안되는디?
cout << "\a";
return 0;
}
#include <iostream> //cout, cin, endl, ...
#include <cstdio> // printf
int main()
{
using namespace std;
int x;
cin >> x ;
cout << "Your input is" << x <<endl;
return 0;
}
!질문
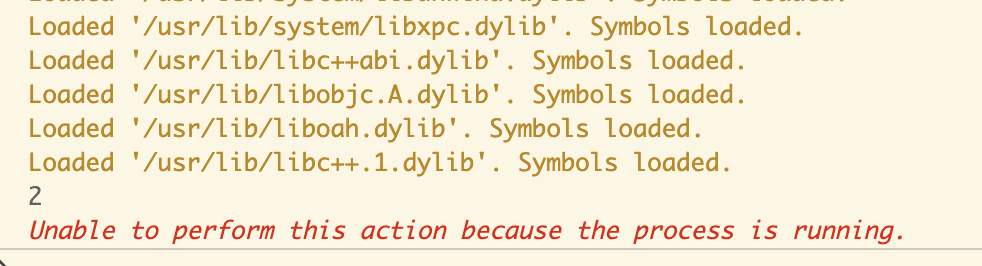

왜 Input이 안먹히는지용?
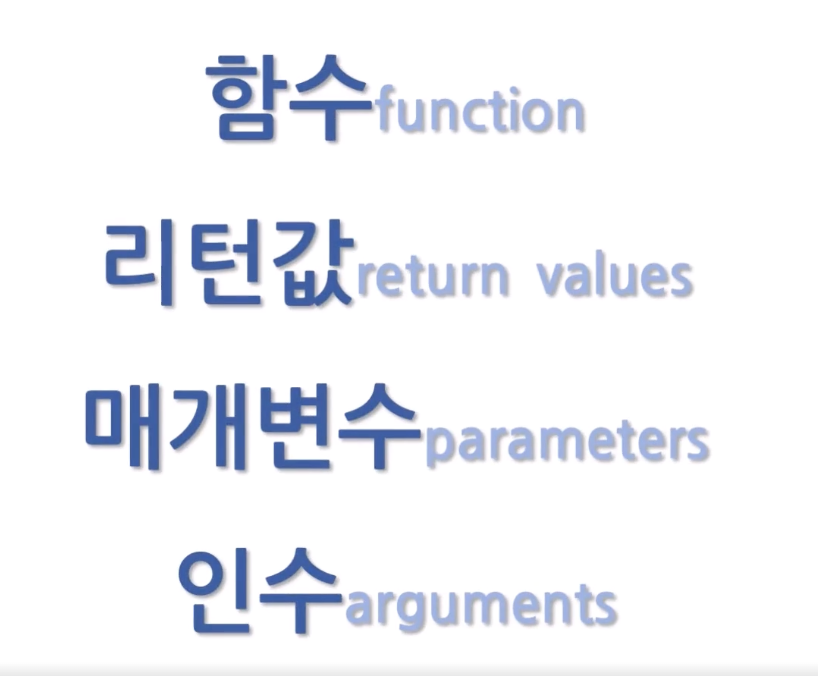
한 가지 패턴이 여러번 반복되면 함수로 대체할 수 있음
#include <iostream> //cout, cin, endl, ...
#include <cstdio> // printf
using namespace std;
int main()
{
cout << 1 + 2 <<endl;
cout << 3 + 4 <<endl;
cout << 8 + 13 <<endl;
return 0;
}
#include <iostream> //cout, cin, endl, ...
#include <cstdio> // printf
using namespace std;
int addTwoNumbers(int num_a, int num_b)
{
int sum = num_a + num_b;
return sum;
}
int main()
{
cout << addTwoNumbers(1, 2) <<endl;
cout << addTwoNumbers(3, 4) <<endl;
cout << addTwoNumbers(8, 13) <<endl;
return 0;
}
나도 저 아래 창 만들고 싶음..
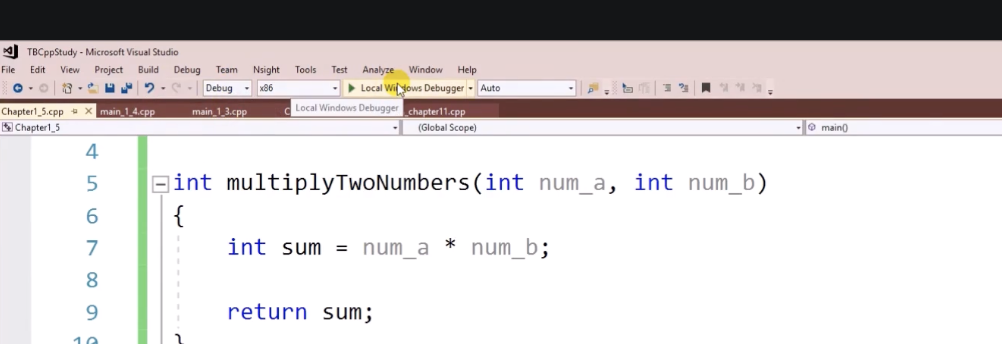
#include <iostream> //cout, cin, endl, ...
#include <cstdio> // printf
using namespace std;
// return할 게 없어서 void
void printHelloWorld()
{
cout << "Hello World" <<endl;
return;
// 어떠한 경우에도 실행되지 않는 코드
cout << "Hello World 2" <<endl;
}
int main()
{
printHelloWorld();
return 0;
}
#include <iostream> //cout, cin, endl, ...
#include <cstdio> // printf
using namespace std;
// return할 게 없어서 void
void addTwoNumber(int a, int b)
{
//return a + b;
}
int main()
{
//int sum = addTwoNumber(1, 2);
addTwoNumber(1, 2);
return 0;
}
함수도 함수를 부르는 예제
함수 안에서 함수를 부를 수는 없음
void addTwoNumber(int a, int b)
{
//return a + b;
void print()
{
cout << "Hello" << endl;
}
}
연습 문제
숫자 두 개를 입력 받아서 더하기를 출력하는 프로그램 만들기
-> 함수로 쪼개서 사용자로부터 여러번 입력 받고 여러번 출력받는 프로그램 만들어보기
'C++' 카테고리의 다른 글
선언과 정의의 분리/ 헤더 파일 만들기 (0) | 2024.03.08 |
---|---|
[VS code 오류]Unable to perform this action because the process is running. (0) | 2024.03.08 |
지역 범위 / 연산자 / Formatting (0) | 2024.03.07 |
키워드 식별자 이름 짓기 (1) | 2024.03.06 |
프로그램의 구조 (0) | 2024.03.06 |